Our OWN malloc:
copied from here:
https://danluu.com/malloc-tutorial/
Let’s write a malloc and see how it works with existing programs!
This tutorial is going to assume that you know what pointers are, and that you know enough C to know that
*ptr
dereferences a pointer, ptr->foo
means (*ptr).foo
, that malloc is used to dynamically allocate space, and that you’re familiar with the concept of a linked list. For a basic intro to C, Pointers on C is one of my favorite books. If you want to look at all of this code at once, it’s available here.Preliminaries aside, malloc’s function signature is
void *malloc(size_t size);
It takes as input a number of bytes and returns a pointer to a block of memory of that size.There are a number of ways we can implement this. We’re going to arbitrarily choose to use the sbrk syscall. The OS reserves stack and heap space for processes and sbrk lets us manipulate the heap.
sbrk(0)
returns a pointer to the current top of the heap. sbrk(foo)
increments the heap size by foo
and returns a pointer to the previous top of the heap.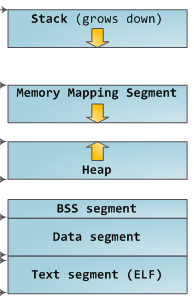
If we want to implement a really simple malloc, we can do something like
#include <assert.h>
#include <string.h>
#include <sys/types.h>
#include <unistd.h>
void *malloc(size_t size) {
void *p = sbrk(0);
void *request = sbrk(size);
if (request == (void*) -1) {
return NULL; // sbrk failed.
} else {
assert(p == request); // Not thread safe.
return p;
}
}
When a program asks malloc for space, malloc asks sbrk to increment the heap size and returns a pointer to the start of the new region on the heap. This is missing a technicality, that malloc(0)
should either return NULL
or another pointer that can be passed to free without causing havoc, but it basically works.But speaking of free, how does free work? Free’s prototype is
void free(void *ptr);
When free is passed a pointer that was previously returned from malloc, it’s supposed to free the space. But given a pointer to something allocated by our malloc, we have no idea what size block is associated with it. Where do we store that? If we had a working malloc, we could malloc some space and store it there, but we’re going to run into trouble if we need to call malloc to reserve space each time we call malloc to reserve space.A common trick to work around this is to store meta-information about a memory region in some space that we squirrel away just below the pointer that we return. Say the top of the heap is currently at
0x1000
and we ask for 0x400
bytes. Our current malloc will request 0x400
bytes from sbrk
and return a pointer to 0x1000
. If we instead save, say, 0x10
bytes to store information about the block, our malloc would request 0x410
bytes from sbrk
and return a pointer to 0x1010
, hiding our 0x10
byte block of meta-information from the code that’s calling malloc.our own sizeof:
#define my_sizeof(type) (char *)(&type+1)-(char*)(&type) int main() { double x; printf ( "%d" , my_sizeof(x)); getchar (); return 0; } |
No comments:
Post a Comment